Building modern applications means choosing the right tools for the job. When it comes to the difference between React and React Native, many developers get confused because the names are so similar. In this post, your go-to guide for React vs React Native and React Native vs. React, we’ll cover:
- What React and React Native are
- Their fundamental differences (in prose)
- Typical use cases for each
- How do they compare in performance?
- Tips for choosing between them
By the end, you’ll understand not only React and React Native individually, but also the key distinctions that drive the decision to build a web app with React or a mobile app with React Native.
1. What Is React?
Whether you’re exploring the diff between React and React Native, comparing React vs. React Native, or React Native vs. React, it’s important to know that React and React Native share their core, but React itself is a JavaScript library for building user interfaces on the web. Developed at Facebook in 2013, its main features are
- Component-based architecture: Build encapsulated UI pieces that manage their state (e.g., <Button> or <TodoList>).
- Declarative rendering: Describe “what” your UI looks like for any application state; React updates the DOM.
- Virtual DOM: A lightweight in-memory representation of the real DOM that minimizes costly browser updates.
- Ecosystem: Integrates with React Router, Redux/Recoil for state, styled-components or CSS Modules for styling, and a vast library community.
React powers countless websites and single-page apps where HTML, CSS, and browser APIs are the delivery medium.
2. What Is React Native?
React Native brings React’s component model to native mobile apps on iOS and Android. Released by Facebook in 2015, its defining traits include
- Native rendering: React Native components like <View> and <Text> map directly to platform UI widgets (UIView on iOS, Android View).
- JavaScript bridge: Your JS logic runs in a separate thread and communicates over a bridge to render native components.
- Styling with JS: Styles are defined in JavaScript via StyleSheet.create(), leveraging Flexbox for layout without cascading CSS.
- Access to device APIs: camera, GPS, push notifications, and more through built-in and community “native modules.”
- Hot/Fast Refresh: Quickly see code changes in simulators or devices without full rebuilds.
React Native lets you reuse JavaScript logic across web and mobile, but the UI layer is truly native.
Recommended Reads: Next.js vs Remix: Choosing the Right Framework for your project
3. Key Differences (Without Tables)
1. Rendering Layers
React outputs standard HTML elements (like <div> and <button>) into the browser’s DOM. React Native, by contrast, never uses HTML—instead, it invokes native UI components (<View>, <Text>, etc.) on iOS and Android.
2. Styling Approach
In React, you style with CSS—whether via external stylesheets, CSS Modules, or CSS-in-JS libraries. React Native uses JavaScript objects (the StyleSheet API), following Flexbox rules but without selectors or cascading styles.
3. Navigation & Routing
Web apps rely on libraries such as React Router or frameworks like Next.js to manage URL-based routing. Mobile apps built with React Native use navigation stacks and tabs via React Navigation or React Native Navigation, simulating native transitions and screen stacks.
4. Platform APIs
React taps directly into browser capabilities (Fetch, WebSockets, Geolocation). React Native accesses device hardware (camera, sensors, push notifications) through native modules that bridge to platform SDKs.
5. Build & Deployment
React apps are bundled with tools like Webpack or Vite and deployed as static files to a server or CDN. React Native relies on Metro Bundler plus Xcode (iOS) or Android Studio/Gradle to produce installable IPA/APK packages.
6. Animation & Gesture Handling
On the web, you might use CSS transitions or libraries like Framer Motion. In React Native, you lean on the built-in Animated API or Reanimated, combined with gesture handlers for smooth, native-driven animations.
7. Code Reuse
While both share JavaScript logic—hooks, utilities, state management—their UI layers differ. However, with tools like React Native Web, you can strive for maximum reuse of component logic and even some UI code across web and mobile.
8. Ecosystem Focus
React’s ecosystem is vast and web-centric, covering everything from SEO-friendly frameworks to chart libraries. React Native’s community is growing around mobile needs—Expo for rapid prototyping, native device plugins, and performance tools like Hermes.
4. Use Cases
When to Choose React (Web)
- Rich browser apps: dashboards, CMS, content sites, and online marketplaces.
- Progressive Web Apps (PWAs): Web code plus service workers for offline support.
- SEO-critical pages: Server-side rendering (Next.js) for indexable content.
When to Choose React Native (Mobile)
- Cross-platform mobile apps: Share business logic across iOS & Android.
- Access to device hardware: GPS, camera, sensors, notifications.
- Performance-sensitive UIs need native animations and smooth gestures.
- You can also use React Native Web to target browsers, iOS, and Android from a single codebase—ideal for maximum reuse.
5. Performance Comparison
1. Startup Time
Web apps depend on bundle size and network latency, whereas native apps often launch faster once installed.
2. UI Updates
In React, virtual DOM diffing can cause browser reflows, while React Native sends update commands through the JavaScript bridge to modify native UI components.
3. Animations
Browser animations can drop frames under heavy load; React Native’s native-driven animations (especially with Reanimated) tend to maintain a smooth 60 fps.
4. Bundle Size & Memory
Web bundles can be split and lazy-loaded; React Native apps can optimize with inline requires and engines like Hermes, though overall memory usage may be higher.
5. Optimization Tips:
On the web, use React.lazy() with Suspense for code-splitting, and wrap pure components using React. memo for performance optimization, and evaluate bundle sizes using webpack-bundle-analyzer.
Mobile: Turn on Hermes for Android, use inline requires, offload heavy tasks to native modules, and minimize JS-to-native bridge calls.
Recommended Reads: React vs Next.js, which framework is best for seo
6. Sharing & Migration Strategies
Thinking of offering both web and mobile? Follow these steps:
- Isolate business logic: Move hooks, API clients, and utilities into shared packages.
- Create a UI abstraction: Build components that wrap platform-specific primitives—then swap <div> for <View> under the hood.
- Separate navigation layers: Utilize React Router for web applications and React Navigation for mobile platforms.
- Translate styles: Convert CSS into StyleSheet objects, mindful of platform differences (e.g., shadows on iOS vs. elevation on Android).
- With a well-modularized codebase, you can reach 70–80% reuse between web and mobile.
Here are the pros and cons of React and React Native:
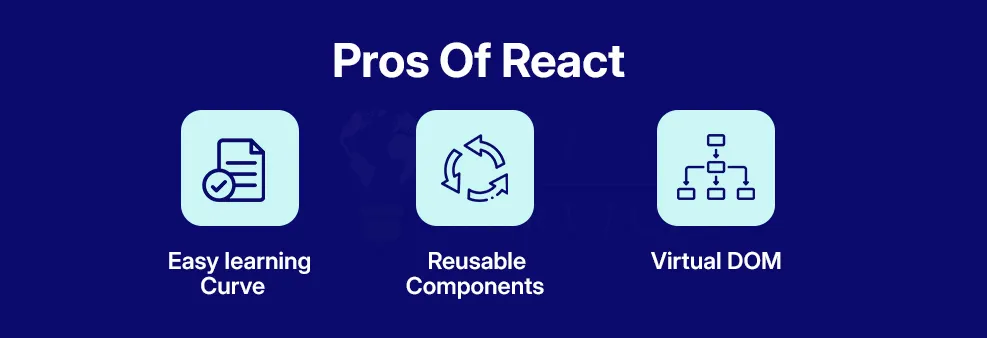
1. React (for Web Development)
Pros of React:
- Reusable Components: Encourages modular design, which saves time and improves maintainability.
- The Virtual DOM enhances performance by reducing direct interactions with the actual DOM.
- Strong Community Support: Vast ecosystem, lots of libraries, tools, and tutorials.
- SEO-Friendly: Can be optimized for search engines using server-side rendering (e.g., with Next.js).
- One-Way Data Binding: Provides improved control over data flow and simplifies debugging.
- Fast Rendering: Efficient updates to the user interface.
Cons of React:
- Learning Curve: JSX and the concept of states/props may be confusing for beginners.
- Constant updates can make it challenging to stay current.
- Poor Documentation for Some Libraries: Community packages may lack sufficient documentation.
- Boilerplate Code: Setting up a React project from scratch can be complex without tools like Create React App.
React Native (for Mobile App Development)
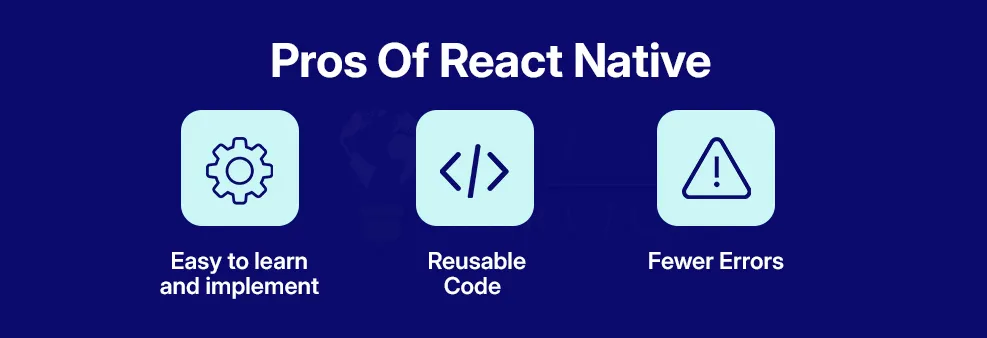
Pros of React Native:
- Cross-Platform Development: Create Android and iOS apps using a single codebase.
- Hot reloading enables developers to instantly observe modifications without requiring a complete application rebuild.
- Delivers performance close to native apps by leveraging native UI components
- Large Community: Plenty of libraries, tools, and community support.
- Cost-Effective: Saves both development time and resources by eliminating the need to build separate native applications.
Cons of React Native:
- Not Fully Native: Some features still require native code (Java/Kotlin for Android, Swift/Obj-C for iOS).
- Third-Party Library Dependency: Many essential features rely on community-built libraries.
- Performance Limitations: Not as fast as purely native apps for complex tasks (e.g., gaming, heavy animations).
- The integration of JavaScript with native code can complicate the debugging process.
Conclusion
- React excels at SEO-friendly, component-driven web UIs using HTML/CSS and browser APIs.
- React Native takes React’s core concepts and applies them to truly native mobile experiences, with access to device hardware and native performance.
When weighing the difference between React and React Native, or comparing React vs. React Native (and even React Native vs. React), the rule of thumb is simple: choose React when you’re targeting browsers, and opt for React Native when you need standalone iOS/Android apps. And because both frameworks share so much under the hood, thanks to React and React Native using the same component model, you can still reuse a large chunk of your JavaScript logic. No matter which path you choose, you’ll be building on a mature, vibrant ecosystem with powerful tooling and community support.